Use Fstack-protector-all (stack Canaries For Mac
- Use Fstack-protector-all (stack Canaries For Mac Pro
- Use Fstack-protector-all (stack Canaries For Macbook Pro
- Use Fstack-protector-all (stack Canaries For Mac)
Code Quality and Build Settings for iOS Apps Making Sure that the App Is Properly Signed Overview Code signing your app assures users that the app has a known source and hasn't been modified since it was last signed. Before your app can integrate app services, be installed on a device, or be submitted to the App Store, it must be signed with a certificate issued by Apple. For more information on how to request certificates and code sign your apps, review the You can retrieve the signing certificate information from the application's.app file with Codesign is used to create, check, and display code signatures, as well as inquire into the dynamic status of signed code in the system. After you get the application's.ipa file, re-save it as a ZIP file and decompress the ZIP file. Navigate to the Payload directory, where the application's.app file will be. Execute the following codesign command. $ gobjdump -stabs -dwarf TargetApp In archive MyTargetApp: armv5te: file format mach-o-arm aarch64: file format mach-o-arm64 Gobjdump is part of and can be installed on macOS via Homebrew.
Dynamic Analysis Dynamic analysis is not applicable for finding debugging symbols. Remediation Make sure that debugging symbols are stripped when the application is being built for production. Stripping debugging symbols will reduce the size of the binary and increase the difficulty of reverse engineering.
To strip debugging symbols, set Strip Debug Symbols During Copy to 'YES' via the project's build settings. A proper is possible because the system doesn't require any symbols in the application binary. Finding Debugging Code and Verbose Error Logging Overview To speed up verification and get a better understanding of errors, developers often include debugging code, such as verbose logging statements (using NSLog, println, print, dump, and debugPrint) about responses from their APIs and about their application's progress and/or state. Furthermore, there may be debugging code for 'management-functionality,' which is used by developers to set the application's state or mock responses from an API. Reverse engineers can easily use this information to track what's happening with the application.
Therefore, debugging code should be removed from the application's release version. Static Analysis You can take the following static analysis approach for the logging statements:. Import the application's code into Xcode.
Search the code for the following printing functions: NSLog, println, print, dump, debugPrint. When you find one of them, determine whether the developers used a wrapping function around the logging function for better mark up of the statements to be logged; if so, add that function to your search. For every result of steps 2 and 3, determine whether macros or debug-state related guards have been set to turn the logging off in the release build. Please note the change in how Objective-C can use preprocessor macros.
# ifdef DEBUG // Debug-only code # endif The procedure for enabling this behavior in Swift has changed: you need to either set environment variables in your scheme or set them as custom flags in the target's build settings. Please note that the following functions (which allow you to determine whether the app was built in the Swift 2.1. Release-configuration) aren't recommended, as Xcode 8 and Swift 3 don't support these functions:. isDebugAssertConfiguration. isReleaseAssertConfiguration. isFastAssertConfiguration. Depending on the application's setup, there may be more logging functions.
For example, when is used, static analysis is a bit different. For the 'debug-management' code (which is built-in): inspect the storyboards to see whether there are any flows and/or view-controllers that provide functionality different from the functionality the application should support. This functionality can be anything from debug views to printed error messages, from custom stub-response configurations to logs written to files on the application's file system or a remote server. Dynamic Analysis Dynamic analysis should be executed on both a simulator and a device because developers sometimes use target-based functions (instead of functions based on a release/debug-mode) to execute the debugging code. Run the application on a simulator and check for output in the console during the app's execution. Attach a device to your Mac, run the application on the device via Xcode, and check for output in the console during the app's execution in the console.
For the other 'manager-based' debug code: click through the application on both a simulator and a device to see if you can find any functionality that allows an app's profiles to be pre-set, allows the actual server to be selected or allows responses from the API to be selected. Remediation As a developer, incorporating debug statements into your application's debug version should not be a problem if you realize that the debugging statements should never. be present in the application's release version or. end up in the application's release configuration.
In Objective-C, developers can use preprocessor macros to filter out debug code. # if DEBUGLOGGING // Debug-only code # endif Testing Exception Handling Overview Exceptions often occur after an application enters an abnormal or erroneous state. Testing exception handling is about making sure that the application will handle the exception and get into a safe state without exposing any sensitive information via its logging mechanisms or the UI. Bear in mind that exception handling in Objective-C is quite different from exception handling in Swift.

Bridging the two approaches in an application that is written in both legacy Objective-C code and Swift code can be problematic. Exception handling in Objective-C Objective-C has two types of errors: NSException NSException is used to handle programming and low-level errors (e.g., division by 0 and out-of-bounds array access). An NSException can either be raised by raise or thrown with @throw. Unless caught, this exception will invoke the unhandled exception handler, with which you can log the statement (logging will halt the program). @catch allows you to recover from the exception if you're using a @try- @catch-block. FunctionThatThrows //In this case the value of x is nil in case of an error. Use the try!
Expression to assert that the error won't occur. Static Analysis Review the source code to understand how the application handles various types of errors (IPC communications, remote services invocation, etc.). The following sections list examples of what you should check for each language at this stage. $ unzip DamnVulnerableiOSApp.ipa $ cd Payload/DamnVulnerableIOSApp.app $ otool -hv DamnVulnerableIOSApp DamnVulnerableIOSApp (architecture armv7): Mach header magic cputype cpusubtype caps filetype ncmds sizeofcmds flags MHMAGIC ARM V7 0x00 EXECUTE 38 4292 NOUNDEFS DYLDLINK TWOLEVEL WEAKDEFINES BINDSTOWEAK PIE DamnVulnerableIOSApp (architecture arm64): Mach header magic cputype cpusubtype caps filetype ncmds sizeofcmds flags MHMAGIC64 ARM64 ALL 0x00 EXECUTE 38 4856 NOUNDEFS DYLDLINK TWOLEVEL WEAKDEFINES BINDSTOWEAK PIE. stack canary.
$ otool -Iv DamnVulnerableIOSApp grep release 0x0045b7dc 83156 cxaguardrelease 0x0045fd5c 83414 objcautorelease 0x0045fd6c 83415 objcautoreleasePoolPop 0x0045fd7c 83416 objcautoreleasePoolPush 0x0045fd8c 83417 objcautoreleaseReturnValue 0x0045ff0c 83441 objcrelease SNIP With idb IDB automates the processes of checking for stack canary and PIE support. Select the target binary in the IDB GUI and click the 'Analyze Binary' button. Checking for Weaknesses in Third Party Libraries Overview iOS applications often make use of third party libraries. These third party libraries accelerate development as the developer has to write less code in order to solve a problem.
There are two categories of libraries:. Libraries that are not (or should not) be packed within the actual production application, such as OHHTTPStubs used for testing.
Libraries that are packed within the actual production application, such as Alomofire. These libraries can have the following two classes of unwanted side-effects:. A library can contain a vulnerability, which will make the application vulnerable. A good example is AFNetworking version 2.5.1, which contained a bug that disabled certificate validation.
This vulnerability would allow attackers to execute man-in-the-middle attacks against apps that are using the library to connect to their APIs. A library can use a license, such as LGPL2.1, which requires the application author to provide access to the source code for those who use the application and request insight in its sources. In fact the application should then be allowed to be redistributed with modifications to its source code.
This can endanger the intellectual property (IP) of the application. Note: there are two widely used package management tools: Carthage and CocoaPods. Please note that this issue can hold on multiple levels: When you use webviews with JavaScript running in the webview, the JavaScript libraries can have these issues as well.
The same holds for plugins/libraries for Cordova, React-native and Xamarin apps. Static Analysis Detecting vulnerabilities of third party libraries In order to ensure that the libraries used by the apps are not carrying vulnerabilities, one can best check the dependencies installed by CocoaPods or Carthage.
In case CocoaPods is used for managing third party dependencies, the following steps can be taken to analyse the third party libraries for vulnerabilities:. At the root of the project, where the Podfile is located, execute the following commands. $ sudo gem install CocoaPods-dependencies $ pod dependencies. The result of the steps above can now be used as input for searching different vulnerability feeds for known vulnerabilities.
Use Fstack-protector-all (stack Canaries For Mac Pro
Note:. If the developer packs all dependencies in terms of its own support library using a.podspec file, then this.podspec file can be checked with the experimental CocoaPods podspec checker. If the project uses CocaoPods in combination with Objective-C, SourceClear can be used.
Using CocoaPods with http based links instead of https might allow for man-in-the-middle attacks during the download of the dependency, which might allow the attacker to replace (parts of) the library you download with other content. Therefore: always use https. In case Carthage is used for third party dependencies, then the following steps can be taken to analyse the third party libraries for vulnerabilities:.
At the root of the project, where the Cartfile is located, type. $ brew install carthage $ carthage update -platform iOS. Check the Cartfile.resolved for actual versions used and inspect the given libraries for known vulnerabilities.
Use Fstack-protector-all (stack Canaries For Macbook Pro
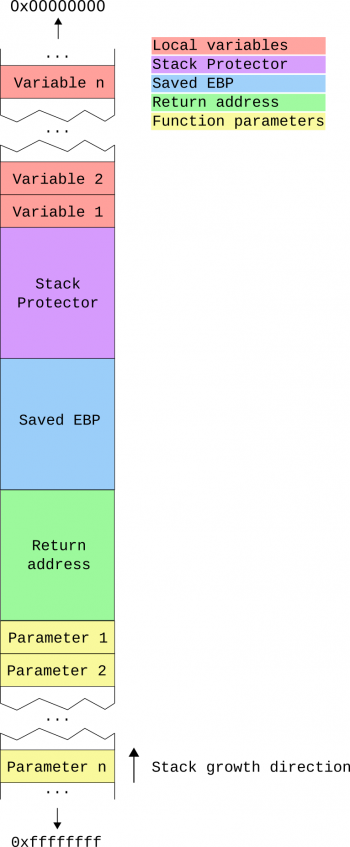
Note, at the time of writing of this chapter, there is no automated support for Carthage based dependency analysis known to the authors. When a library is found to contain vulnerabilities, then the following reasoning applies:. Is the library packaged with the application?
Then check whether the library has a version in which the vulnerability is patched. If not, check whether the vulnerability actually affects the application. If that is the case or might be the case in the future, then look for an alternative which provides similar functionality, but without the vulnerabilities. Is the library not packaged with the application?
See if there is a patched version in which the vulnerability is fixed. If this is not the case, check if the implications of the vulnerability for the build-proces. Could the vulnerability impede a build or weaken the security of the build-pipeline? Then try looking for an alternative in which the vulnerability is fixed. In case frameworks are added manually as linked libraries:.
Open the xcodeproj file and check the project properties. Go to the tab 'Build Phases' and check the entries in 'Link Binary With Libraries' for any of the libraries. See earlier sections on how to obtain similar information using.
In the case of copy-pasted sources: search the headerfiles (in case of using Objective-C) and otherwise the Swift files for known methodnames for known libraries. Lastly, please note that for hybrid applications, one will have to check the JavaScript dependencies with RetireJS. Similarly for Xamarin, one will have to check the C# dependencies.
Use Fstack-protector-all (stack Canaries For Mac)
Detecting the licenses used by the libraries of the application In order to ensure that the copyright laws are not infringed, one can best check the dependencies installed by CocoaPods or Carthage. When the application sources are available and CocoaPods is used, then execute the following steps to get the different licenses:. At the root of the project, where the Podfile is located, type. $ brew install carthage $ carthage update -platform iOS. The sources of each of the dependencies have been downloaded to Carthage/Checkouts folder in the project. Here you can find the license for each of the libraries in their respective folder. When a library contains a license in which the app's IP needs to be open-sourced, check if there is an alternative for the library which can be used to provide similar functionalities.
Note: In case of a hybrid app, please check the build-tools used: most of them do have a license enumeration plugin to find the licenses being used. Dynamic Analysis The dynamic analysis of this section comprises of two parts: the actual license verification and checking which libraries are involved in case of missing sources. It need to be validated whether the copyrights of the licenses have been adhered to. This often means that the application should have an about or EULA section in which the copy-right statements are noted as required by the license of the third party library.
When no source-code is available for library analysis, you can find some of the frameworks being used with otool and MobSF. After you obtain the library and Clutched it (e.g. Download quake 2 for mac. Removed the DRM), you can run oTool with at the root of the directory. $./class-dump -r References OWASP Mobile Top 10 2016. M7 - Poor Code Quality - OWASP MASVS. V7.1: 'The app is signed and provisioned with a valid certificate.' .
V7.4: 'Debugging code has been removed, and the app does not log verbose errors or debugging messages.' .
V7.5: 'All third party components used by the mobile app, such as libraries and frameworks, are identified, and checked for known vulnerabilities.' . V7.6: 'The app catches and handles possible exceptions.' . V7.7: 'Error handling logic in security controls denies access by default.'
. V7.9: 'Free security features offered by the toolchain, such as byte-code minification, stack protection, PIE support and automatic reference counting, are activated.'
CWE. CWE-937 - OWASP Top Ten 2013 Category A9 - Using Components with Known Vulnerabilities Tools. OWASP Dependency Checker( Dependency Checker'). Go.